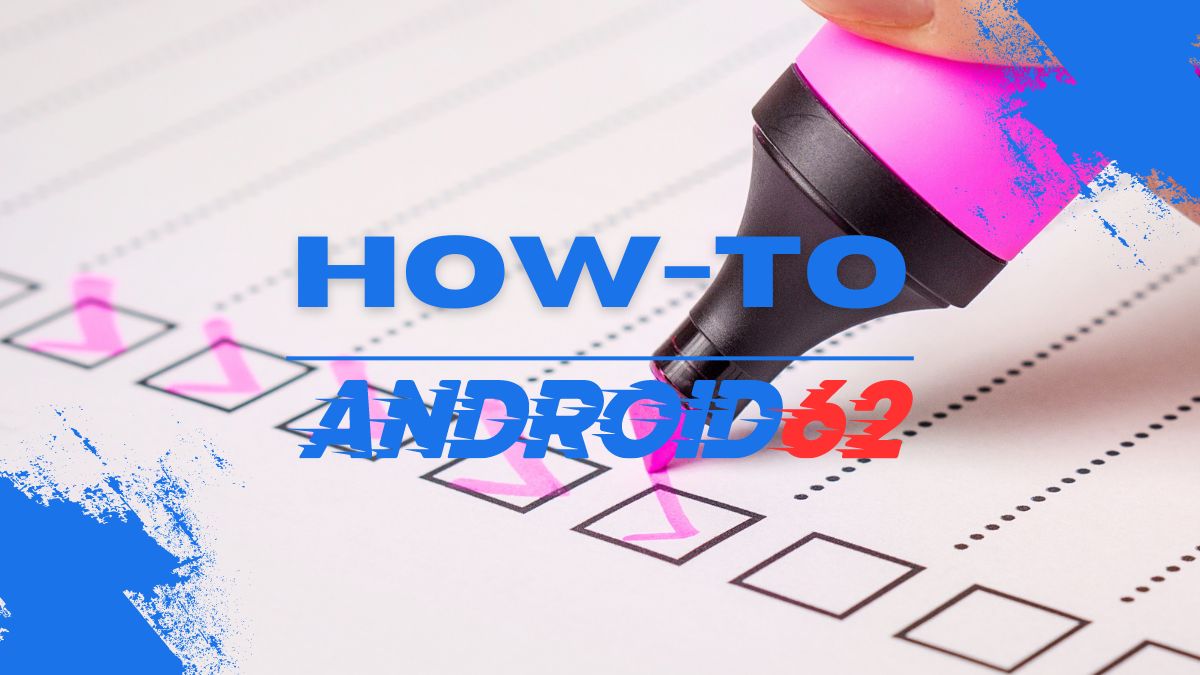
Introduction
In Swift, an array is a collection of values that are stored in an ordered list. If you are working with arrays in Swift, you may need to determine the length of an array at some point. Knowing how to get the length of an array is a fundamental skill in programming. In this article, we will explore various methods to get the length of an array in Swift.
Using the count Property
The most common and straightforward way to get the length of an array in Swift is by using the count property. The count property returns the number of elements in the array.
“`swift
let myArray = [1, 2, 3, 4, 5]
let arrayLength = myArray.count
print(“The length of the array is: \(arrayLength)”)
“`
Using the isEmpty Property
Another way to determine the length of an array is by using the isEmpty property. The isEmpty property returns a Boolean value indicating whether the array is empty or not.
“`swift
let myArray = [1, 2, 3, 4, 5]
if myArray.isEmpty {
print(“The array is empty”)
} else {
print(“The array is not empty”)
print(“The length of the array is: \(myArray.count)”)
}
“`
Using the endIndex Property
The endIndex property returns the index after the last element of the collection, which can be used to calculate the length of an array.
“`swift
let myArray = [1, 2, 3, 4, 5]
let arrayLength = myArray.endIndex
print(“The length of the array is: \(arrayLength)”)
“`
Using the distance(from:to:) Method
You can also use the distance(from:to:) method to calculate the length of an array by finding the distance between the startIndex and endIndex.
“`swift
let myArray = [1, 2, 3, 4, 5]
let arrayLength = myArray.distance(from: myArray.startIndex, to: myArray.endIndex)
print(“The length of the array is: \(arrayLength)”)
“`
Using the withUnsafeBufferPointer Method
The withUnsafeBufferPointer method allows you to access the underlying buffer of the array, which can be used to calculate the length of the array.
“`swift
let myArray = [1, 2, 3, 4, 5]
let arrayLength = myArray.withUnsafeBufferPointer { buffer in buffer.count }
print(“The length of the array is: \(arrayLength)”)
“`
Using the withContiguousStorageIfAvailable Method
The withContiguousStorageIfAvailable method allows you to access the array’s contiguous storage if it’s available, which can be used to calculate the length of the array.
“`swift
let myArray = [1, 2, 3, 4, 5]
if let buffer = myArray.withContiguousStorageIfAvailable({ $0 }) {
let arrayLength = buffer.baseAddress!.distance(to: buffer.baseAddress! + buffer.count)
print(“The length of the array is: \(arrayLength)”)
}
“`
Conclusion
Getting the length of an array is a fundamental operation when working with arrays in Swift. In this article, we have discussed various methods to calculate the length of an array, such as using the count property, isEmpty property, endIndex property, distance(from:to:) method, withUnsafeBufferPointer method, and withContiguousStorageIfAvailable method. Each of these methods has its own advantages and use cases, so choose the method that best suits your needs. Understanding how to get the length of an array will help you write more efficient and optimized code in your Swift projects.