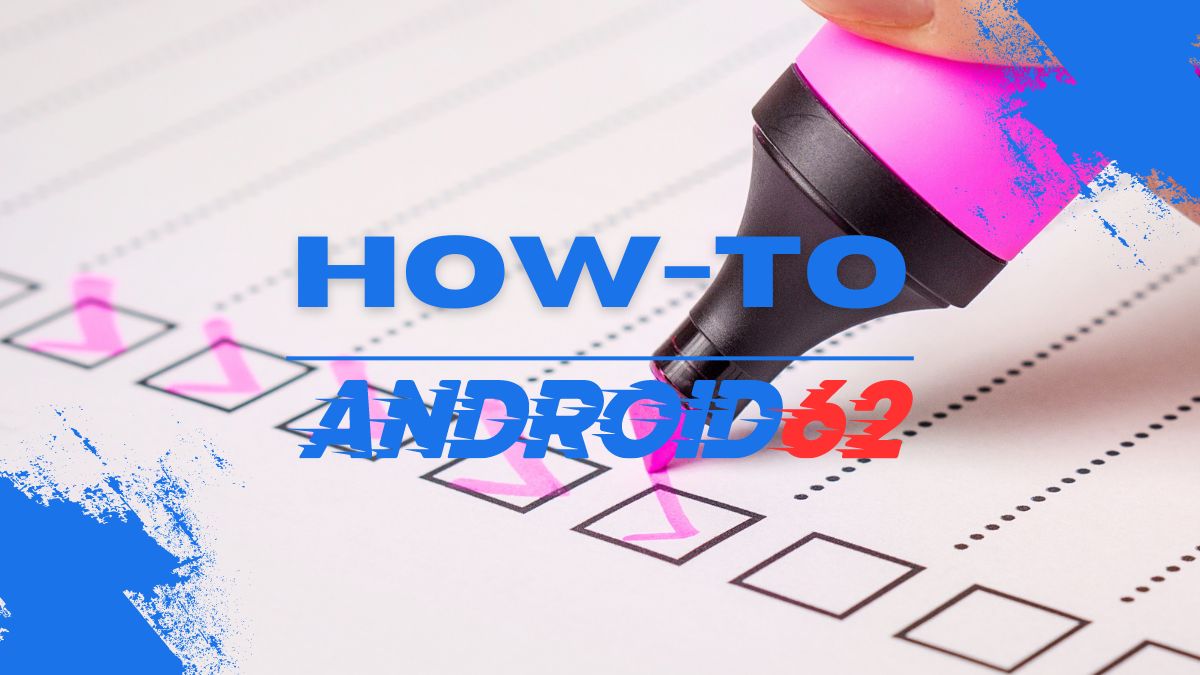
Java is a robust programming language that is widely used for web and application development. One of its fundamental features for handling errors is the exception handling mechanism. In this article, we will delve into the intricacies of throwing exceptions in Java, providing a comprehensive guide that covers everything from basic definitions to practical examples, ensuring a deep understanding of how to effectively implement exception handling in your Java applications.
Understanding Exceptions in Java
Exceptions are events that disrupt the normal flow of a program’s execution. In Java, exceptions are objects that represent an error or an unexpected condition that occurs during runtime. When an exception is thrown, it creates a pathway for the program to handle the error gracefully.
Types of Exceptions:
Java has two main categories of exceptions: checked and unchecked exceptions. Checked exceptions extend from the Exception
class and must be either caught or declared in the method signature. On the other hand, unchecked exceptions, which extend from the RuntimeException
class, do not require explicit handling, allowing developers more flexibility.
Why Throw Exceptions?
Throwing exceptions allows programmers to signal that something unexpected has occurred within a segment of code. By effectively throwing exceptions, developers can prevent the application from crashing and instead redirect the flow of control to an error-handling mechanism.
Advantages of Throwing Exceptions:
- Separation of Error Handling and Regular Code: Exceptions help segregate the error handling logic from the main business logic, leading to cleaner code.
- Code Reusability: Custom exceptions can be reused across various methods or classes.
- Error Propagation: Exceptions can propagate up the call stack, allowing higher-level methods to handle errors centrally.
How to Throw an Exception in Java
In Java, you can throw exceptions using the throw
keyword. This mechanism allows developers to create an instance of an exception class and "throw" it to handle it later in the program flow.
Syntax of Throwing Exceptions:
The basic syntax of throwing an exception in Java is as follows:
throw new ExceptionType("Error Message");
Here, ExceptionType
can be any specific exception such as IOException
, NullPointerException
, etc. The "Error Message" is a string that provides details about the error.
Creating Custom Exceptions
Java allows developers to create custom exceptions to represent specific application-level errors. This feature enhances error handling by allowing for more descriptive and contextual exceptions.
Steps to Create a Custom Exception:
- Create a new class that extends the
Exception
class (for checked exceptions) or theRuntimeException
class (for unchecked exceptions). - Define a constructor that accepts a String message.
- Optionally, you can add additional constructors for different use cases.
Example of a Custom Exception:
public class InvalidInputException extends Exception {
public InvalidInputException(String message) {
super(message);
}
}
Throwing Custom Exceptions
Once you’ve created a custom exception, you can incorporate it seamlessly into your methods. Here’s a practical example showing how to throw a custom exception based on certain conditions.
Example:
public void validateInput(String input) throws InvalidInputException {
if (input == null || input.isEmpty()) {
throw new InvalidInputException("Input cannot be null or empty");
}
}
Catching Exceptions
While throwing exceptions is crucial, catching them is equally important for managing errors effectively. Java uses try-catch blocks to handle exceptions.
Basic Structure of Try-Catch:
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
In this structure, the code wrapped in the try
block is executed, and if an exception occurs, the control is transferred to the corresponding catch
block.
Example of Catching Exceptions:
try {
validateInput(null);
} catch (InvalidInputException e) {
System.out.println("Caught exception: " + e.getMessage());
}
Multiple Catch Blocks
Java allows for multiple catch blocks to handle different types of exceptions that may arise from a single try
block. This flexibility enables developers to provide specific responses for various error types.
Multiple Catch Example:
try {
// Code that may throw different exceptions
} catch (IOException e) {
// Handle IOException
} catch (NullPointerException e) {
// Handle NullPointerException
}
Using Finally Block
The finally
block can be added after the try-catch
blocks, which always executes regardless of whether an exception has been thrown or caught. This block is particularly useful for releasing resources such as file handles or database connections.
Example:
try {
// Code that may throw an exception
} catch (Exception e) {
// Handle exception
} finally {
// Code that will always execute
}
The throws
Keyword
In some cases, it might be more appropriate to declare an exception rather than catching it within a method. The throws
keyword is used in a method declaration to specify the exception types that a method can throw.
Syntax of Using throws
:
public void methodName() throws ExceptionType {
// Code that may throw an exception
}
Once a method declares a throws
clause, it becomes the caller’s responsibility to handle the exception.
Example:
public void readFile() throws IOException {
// Code that may throw IOException
}
Best Practices for Exception Handling
Implementing effective exception handling can significantly improve the reliability and maintainability of your Java applications. Here are some best practices:
1. Catch Specific Exceptions:
Always catch the most specific exceptions possible. This approach allows for better handling processes and clearer code.
2. Do Not Use Exceptions for Control Flow:
Exceptions should not be used as a means for controlling regular program flow. They should only represent error conditions.
3. Log Exception Details:
Logging exceptions helps in debugging and maintaining applications. Always log the stack trace and context of the exception for future reference.
4. Clean Up Resources:
Whether an exception occurs or not, ensure that resources are cleaned up using finally
blocks or try-with-resources construct.
5. Provide Clear Error Messages:
Ensure the messages associated with thrown exceptions are clear and informative, aiding in troubleshooting.
Using the try-with-resources
Statement
In Java 7 and above, the try-with-resources
statement provides an efficient way to handle resources. This structure automatically handles resource cleanup when the try block finishes, whether normally or due to an exception.
Example of Try-With-Resources:
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
// Read from the file
} catch (IOException e) {
e.printStackTrace();
}
// No need for finally block to close resources
Conclusion
Managing exceptions in Java is essential for building resilient applications. By understanding how to throw, catch, and create custom exceptions, developers can create software that gracefully handles errors and improves user experience. Through careful implementation of exception handling best practices, you can enhance the robustness and maintainability of your Java applications. This comprehensive guide has provided a complete overview of how to throw exceptions in Java, equipping you with the knowledge needed to implement effective error handling in your projects.