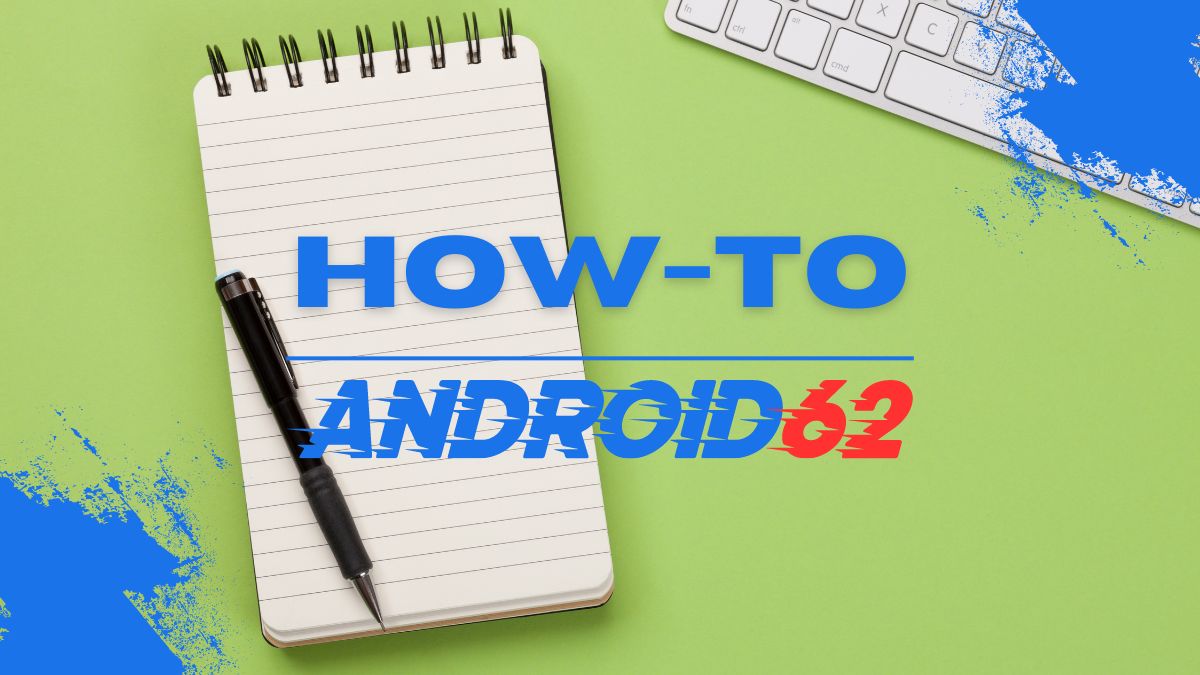
When working with input/output in C++, the getline
function is an invaluable tool for reading strings from various input streams. Whether dealing with text files or console input, getline
helps manage user-friendly input by capturing entire lines, including whitespaces, ensuring better data integrity. This article explores its usage, syntax, and underlying mechanisms in detail.
Understanding Getline in C++
getline
is a part of the C++ Standard Library, which allows for reading lines of text into a string variable. This feature is particularly useful when you want to capture user inputs that contain spaces, unlike the traditional cin
function, which stops reading at the first whitespace.
Using getline
effectively can improve your programming experience, especially in applications where text data is the main focus. The primary reason developers prefer getline
is its ability to handle user input in a more flexible way than other input methods.
Syntax of Getline
The basic syntax of getline
is as follows:
getline(input_stream, string_variable);
Here, input_stream
is typically std::cin
for console input or an std::ifstream
object for file input. The string_variable
is where the input line will be stored.
Additionally, a third optional parameter allows you to specify a delimiter, which by default is the newline character (\n
). This means that getline
will read input until it encounters this delimiter.
Example Syntax with Delimiter
getline(input_stream, string_variable, delimiter);
This can be useful in scenarios where you need to read data in a specific format, such as CSV files.
How to Include Getline
To use getline
in your C++ program, you need to include the <iostream>
and <string>
headers. Below is the necessary code to get started:
#include <iostream>
#include <string>
Incorporating these headers at the beginning of your program gives you access to both input/output functions and the string type defined in the C++ Standard Library.
Basic Getline Example
To understand how getline
works, let’s look at a simple example that reads a line of text from the user.
#include <iostream>
#include <string>
int main() {
std::string userInput;
std::cout << "Enter a line of text: ";
std::getline(std::cin, userInput);
std::cout << "You entered: " << userInput << std::endl;
return 0;
}
In this example, the program prompts the user to enter a line of text. Once the user inputs their line, it captures the entire string, including spaces, and then outputs the entered information.
Reading Multiple Lines of Input
Using getline
to read multiple lines of input is straightforward and commonly needed in applications dealing with extensive user data. To achieve this, you can utilize a loop.
Example of Reading Until a Specific Condition
#include <iostream>
#include <string>
int main() {
std::string lineInput;
std::cout << "Enter multiple lines of text (type 'exit' to stop):\n";
while (true) {
std::getline(std::cin, lineInput);
if (lineInput == "exit") {
break;
}
std::cout << "You entered: " << lineInput << std::endl;
}
return 0;
}
In this example, a while
loop repeatedly reads lines until the user types "exit." This practical approach allows developers to interactively gather data until a stopping criterion is met.
Using Getline with File Input
Another powerful application of getline
is its capability to read from files. To do this, you need to open a file and utilize an std::ifstream
object. This is especially useful for reading configuration files or batch processing data.
Example of File Input with Getline
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
if (!inputFile) {
std::cerr << "Error opening file." << std::endl;
return 1;
}
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
return 0;
}
In this code snippet, we open a file named "example.txt" and read each line until the end of the file. This method is efficient for processing text files line by line.
Handling Delimiters in Getline
Getline can also be customized with a delimiter, allowing for more nuanced input handling. This can come in handy when working with data formatted in specific ways, such as CSV (Comma-Separated Values).
Example Using a Custom Delimiter
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string data = "John,Doe,30";
std::stringstream ss(data);
std::string token;
while (std::getline(ss, token, ',')) {
std::cout << token << std::endl;
}
return 0;
}
Here, we use a std::stringstream
to simulate a data source. The getline
function extracts strings using the comma as a delimiter, a common occurrence in structured data formats.
Common Issues with Getline
Using getline
effectively can sometimes present challenges, particularly when mixing it with cin
. A common pitfall occurs when attempting to read user input after using cin
for formatted input operations, as cin
leaves a newline character in the buffer.
Example of the Common Pitfall and Solution
#include <iostream>
#include <string>
int main() {
int number;
std::string lineInput;
std::cout << "Enter an integer: ";
std::cin >> number;
std::cin.ignore(); // Clearing the newline character from the input buffer
std::cout << "Now, enter a line of text: ";
std::getline(std::cin, lineInput);
std::cout << "You entered integer: " << number << " and line: " << lineInput << std::endl;
return 0;
}
In this code, the std::cin.ignore()
statement clears the input buffer, allowing getline
to work properly. The function takes care of reading the integer, then clearing unread characters from the buffer to ensure the next input call functions correctly.
Performance Considerations
While getline
is efficient for most use cases, consider performance implications in applications dealing with massive input sizes. The allocation of memory for std::string
can lead to overhead, and it’s essential to manage large datasets with care, potentially using methods such as pre-allocating space or utilizing more performant data structures when necessary.
FAQs About Getline
1. Can I use getline with other types of streams, like sockets?
Yes! getline
can be used with any input stream in C++, making it versatile for different contexts, including sockets or any custom stream class you’ve defined.
2. What should I do if I want to read mixed input types?
If you need to read mixed types (e.g., integers and strings), manage your input carefully, using std::cin
for formats and switching to getline
for strings. However, ensure to handle newline characters effectively between inputs.
3. Does getline handle whitespace in input?
Yes! Unlike cin
, which stops reading input at the first whitespace, getline
reads the entire line, including leading and trailing whitespace, making it suitable for a wide range of applications.
By harnessing the capabilities of getline
, C++ programmers can effectively manage text input from users or files, providing intuitive and user-friendly interfaces while maintaining data integrity in their applications. Understanding its functionality, including the syntax and examples illustrated here, equips developers with the robust tools necessary for effective input handling in C++. This foundational function serves both novice and seasoned developers, enabling them to write clear, maintainable, and efficient code.